Carry Bit vs Overflow Bit | A Comprehensive Guide
The carry bit and overflow bit are two crucial concepts in digital logic and computer arithmetic. Though they sound similar, these two bits have distinct meanings and use cases.
This article will provide a deep dive into the carry bit and overflow bit, explaining their definitions, uses, and the mathematical analysis behind them.
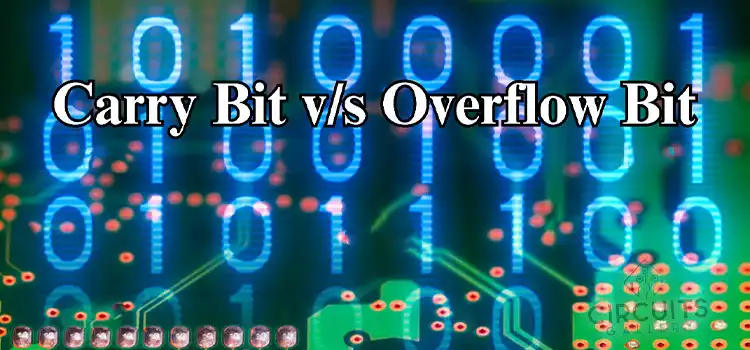
What is Carry Bit and Overflow Bit?
In any arithmetic logic unit (ALU), two key tasks are detecting errors and handling overflows when performing operations like addition, subtraction, etc. The carry bit and overflow bit are specialized flags that allow the ALU to do exactly this.
The carry bit indicates when the result of an arithmetic operation exceeds the available bits in the destination register. It “carries” the extra bit over to the next position.
The overflow bit detects when the signed result of an operation is too large or small to fit in the allocated bits. It signals an error condition that must be handled.
Though related, these two bits have different mathematical meanings. Understanding when each one is triggered is crucial for error-free computations.
Carry Bit: Propagating Overflows
The carry bit is straightforward in theory – it propagates overflows in multi-bit arithmetic. Let’s examine this further.
Consider an 8-bit ALU adding two 8-bit numbers. If the result exceeds 255 (the max for 8 bits), the carry bit is set to 1. This carry is propagated over to the next position in multi-byte arithmetic.
Mathematically, the carry bit can be expressed as:
Carry = (Result >> n) & 1
Here, n is the number of bits available in the destination register.
For example, in an 8-bit ALU:
Carry = (Result >> 8) & 1
This extracts the 9th bit of the result, which indicates if a carry occurred.
The carry bit is used in both signed and unsigned arithmetic as it solely depends on the result exceeding the register capacity.
Overflow Bit: Detecting Signed Overflow
The overflow bit serves a different purpose – it detects signed overflow errors.
Signed numbers use the MSB for the sign bit – 0 for positive, 1 for negative. In signed arithmetic, overflows happen when:
Adding two negative numbers results in a positive outcome
Adding two positive numbers results in a negative outcome
Mathematically, the overflow bit is calculated as:
Overflow=Sign BitXORSign of Operand1XOR(Sign of Operand2)
The XOR operation detects if the signs are inconsistent.
For example, in 8-bit signed arithmetic:
Overflow = (Result [7bit] XOR Op1[7bit] XOR Op2[7bit])
Here, the sign bits are checked for mismatches to identify overflows.
The overflow bit is triggered only in signed arithmetic as unsigned numbers don’t have sign bits.
Key Differences Between Carry and Overflow Bits
Bit | Role | Used in | Formula |
Carry | Propagates excess bits | Unsigned and Signed arithmetic | (Result >> n) & 1 |
Overflow | Detects sign errors | Signed arithmetic only | (Sign of Result) XOR (Sign of Operands) |
While related in the context of arithmetic flags, the carry and overflow bits are fundamentally different:
- The carry bit handles overflow due to the result size exceeding the register capacity.
- The overflow bit specifically checks for sign errors in signed arithmetic.
Understanding this distinction is vital for low-level programming and hardware design. Misusing these flags can lead to hard-to-debug errors and crashes.
Examples of Carry and Overflow
Let’s examine some examples to differentiate the carry and overflow bits:
Unsigned Addition
Case | Example | Binary Representation | Carry Bit | Overflow Bit |
Unsigned addition | 0xFF + 0xFF = 0x1FE | 11111111 + 11111111 = 11111110 | 1 | 0 |
Signed Addition:
Case | Example | Binary Representation | Carry Bit | Overflow Bit |
Signed addition | -128 + -128 = -256 | 10000000 + 10000000 = 11000000 | 1 | 1 |
Signed Addition:
Case | Example | Binary Representation | Carry Bit | Overflow Bit |
Unsigned subtraction | 0x00 – 0xFF = 0xFF | 00000000 – 11111111 = 11111111 | 0 | 0 |
Signed Subtraction:
Case | Example | Binary Representation | Carry Bit | Overflow Bit |
Signed subtraction | 128 – 128 = 0 | 01111111 – 01111111 = 00000000 | 0 | 0 |
These examples demonstrate the nuances between the carry and overflow flags. Both are vital for robust arithmetic implementations.
Hardware Implementation of Carry and Overflow
At the hardware level, how are the carry and overflow bits implemented in an ALU?
- For carry bits, the full-adder circuits used have a dedicated carry-out wire that propagates the carry to the next adder stage. So, overflows automatically generate the carry-out signal.
- For overflow bits, additional logic is needed to check for sign errors in 2’s complement signed arithmetic. This involves examining the sign bits of operands and results for mismatches.
The carry-bit wiring is simpler compared to the combinational logic used for overflow detection. But both are indispensable ALU features for reliable arithmetic.
Applications and Importance
The carry and overflow bits find widespread use across computer arithmetic implementations:
- Processor ALUs: Used to set status flags when arithmetic instructions are executed. Enables branch decisions based on results.
- Hardware Adders: Propagates overflows across multi-byte additions. Detects errors from sign inconsistencies.
- Programming Languages: Used to implement numeric datatypes and operations in software. Sign errors can raise exceptions.
- Embedded Systems: Lightweight processors rely on these flags for arithmetic sanity checks before acting on data.
Though hidden from software, these bits are continuously working in the background to keep arithmetic reliable and error-free. Understanding them provides key insights into the foundations of computer logic.
Frequently Asked Questions (FAQs)
1. What Causes the Carry Bit to Be Set?
Answer: The carry bit is set when the result of an arithmetic operation exceeds the available bits in the destination register. For example, adding two 8-bit numbers each with a value of 200 would result in a 9-bit number, so the carry bit would be set.
2. When Does the Overflow Bit Indicate an Error?
Answer: The overflow bit indicates an error in signed arithmetic when the signs of the operands are inconsistent with the sign of the result. This happens when adding two negative numbers gives a positive result, or when adding two positive numbers gives a negative result.
3. Can the Carry and Overflow Bits Be Set at the Same Time?
Answer: Yes, both the carry bit and overflow bit can be set after a single arithmetic operation. This would occur in a signed addition where the result exceeds the register capacity and the signs of the operands do not match the sign of the result.
To conclude
Mastering the concepts of carry bit and overflow bit will make you a better programmer and computer architect. You gain a deeper appreciation for the elegance of fundamental logic design. Next time you write code for any arithmetic, remember the crucial carry and overflow bits silently ensuring correct results!
Subscribe to our newsletter
& plug into
the world of circuits